The first thing to come in a long line of rants about ants.
In fact, here's the code to post it somewhere if you want:
Wednesday, July 1, 2009
Sunday, June 28, 2009
Development and Design Contracts
I've got a secret and it's kind of embarrassing - I don't normally work with contracts. Ok so I've definitely been in contact with some contracts, just nothing terribly extensive and nothing I've wrote. Today that changes, though, and while there have been times where I've had to learn a lesson or two the hard way the main factor is simply the increase in scope of the projects I've been dealing with. Contingencies are popping up too frequently to ignore, and with the way one job easily breaks down into many sometimes it's hard to know exactly where the onus or responsibility lie for certain aspects of a project. This latest project I speak of involves a number of special issues that need to be addressed ahead of time -
- The fact that the business this site will serve is in a medical field (which will probably mean very stringent stipulations on how certain kinds of information are handled) may require I ensure a lack of liability if certain standards are not met that I've not been made aware of.
- The fact that the design and development of this site may result in patents or copyrights and where the ownership of these properties lie, considering that ideas the client and I have had previous to this project somewhat overlap. How will these be managed in the near future? Do competing patents already exist?
- This project may have the potential to generate publicity. I would like to have my name or my business' name in the mix where possible. I also want to make sure I retain the right to exhibit this work in my portfolio.
With all these things in mind, I got to googling and crawling wikipedia for information about intellectual property, software licenses (to possibly govern the use of software created as a result), and points covered in contracts by either software developers or graphic & web designers.
What I found was nothing less than ...perplexing. Apparently software falls under the protection of both copyright (as literature) and patent - definitely some sort of sign from the universe. Legal precedents also differ from state to state, which encouraged the shift of such matters to a national court, but even at that level there are competing bodies with differing opinions on how to manage these types of patents and whether they should even exist.
This makes the existence of other (somewhat) similar patents sting a bit less.
Anyways, here's a quick rundown of information I found pertaining to design work:
- 10 Contracts for your next Agile Software Project - Agile development is a set of methodologies for managing projects, when you get down to it. These can be applied to other fields, so why can't their contracts? Mainly these address payment plans and product cycles.
- Sample Buyer/Service Provider Agreement Letter - From Elance.
- Good Contract for Web Designers - I really like that this contract breaks down the "additional costs" of all the sometimes unaccounted for tasks that come with but aren't directly related to web design.
- Web Site and Software Contracts - I know nothing about this guy or the quality of his work or representation, but he gives a handy little guide and glossary for dealing with contracts of these natures. :)
- Legalese for Freelancers: Creating a Contract - goodie from the regulars over at FreelanceSwitch. Breaks the process of getting your documents into phases.
Keeping things I read at these sites and others in mind, as well as certain aspects particular to the project, I came up with the following list of bullet points I'd like to cover in my contract (as well as an example of each) -
- Functional definition of project
- Retain the right to use in portfolio
- Payment regardless of use
- Bonuses in penalties as they relate to deadlines
- The providing of documentation (from both parties)
- Ownership/licensing of copyrights and patents
- Procedure for enacting changes to approved designs
- Specific number of included (free) changes
- Shared-risk pricing scheme
- Explicit stating of independent contractor status
- Reimbursement of expenses
- Limited liability (concerning copyrighted materials and medical guidelines)
- Assignment of work - retaining the right to hire other designers
- Reservation of rights - retaining all rights to content not explicitly handled in contract (design sketches, class diagrams, notes, etc)
- Publication - specifying instances when I should be publicly credited with the work, what kinds of uses should be cleared with myself or the client first, etc
...and a couple of others. Once I feel I've enough reliable examples, I'll begin compiling them into one document which I'll then take to a lawyer to review for it's legality and presentation. So far this all looks pretty good to me but I'm sure there's something I'm leaving out, or at the very least that this will be something I'll speak on again in the future.
Labels:
business
Monday, June 15, 2009
Using MonsterDebugger with AsUnit tests
So I've been using two new tools lately to develop my framework - De Monster Debugger and AsUnit. De Monster is a tool that allows for introspection of you running swf through their air front end. All methods and properties are exposed, and can be executed or edited right from their tree interface. You can find it here or watch Lee Brimelow's tutorial about it.
AsUnit is a unit testing framework for actionscript which allows you to run automated test suites on the smallest, indivisible pieces of your code to ensure integrity. In my opinion the real power of unit testing comes from two places - it formalizes the elimination of possible bug causes, (no more sloppy, randomly placed traces or commenting out code), and secondly it's no big deal to run your entire suite of tests every time you compile your swf, so you catch on when bugs in one class effect other classes in ways you didn't expect.
In essence, AsUnit is basically a framework for setting your classes and pieces of code on stilts for further examination, but to achieve this, it's standard practice to encapsulate your classes into the test suites in awkward - yet beneficial (to the unit testing) - ways. Let's examine these.
package com.framework.tests.units {
import asunit.framework.TestSuite;
import com.framework.tests.units.LinkNodeTest;
import com.framework.tests.units.LinkedListTest;
/**
* @author Will Saunders
*
* Unit Test Suite for the framework
*
*/
public class AllTests extends TestSuite {
public function AllTests() {
super();
// Link Node Tests
addTest(new LinkNodeTest("testInstantiated"));
addTest(new LinkNodeTest("testAutoAddChild"));
addTest(new LinkNodeTest("testManualAddChild"));
addTest(new LinkNodeTest("testRemoveChild"));
This is an example TestSuite for AsUnit. Test classes - LinkNodeTest in this case - contain the logic for administering and checking the tests, while TestSuites group all the tests together. If you look closely, however, you'll notice that each of these tests happen independently of one another - one test does not run on the same data objects as the ones before or after because a new instance of LinkNodeTest is established for each test. Furthermore their syntax abstracts the inner parts of the Test classes from us. While this method is mostly always a good thing (for the sake of maintaining granular, "blank slate starts" in the tests), but if you want to look into the tests with Monster Debugger to see what's actually happening or what the data looks like after your tests have run you'll have to jump through a few hoops.
First we will add a lasting reference to the particular instance we'd like to examine more closely. I only set aside one of these at a time and to encourage myself not to get too far away from the point of unit testing. We will also add the necessary classes for the debugger:
package com.framework.tests.units {
import asunit.framework.TestSuite;
import com.framework.tests.units.LinkNodeTest;
import com.framework.tests.units.LinkedListTest;
import nl.demonsters.debugger.MonsterDebugger;
/**
* @author Will Saunders
*
* Unit Test Suite for the framework
*
*/
public class AllTests extends TestSuite {
public var failedTest:LinkedListTest = new LinkedListTest("testRemoveChild");
public var bugger:MonsterDebugger = new MonsterDebugger( this );
public function AllTests() {
super();
// Link Node Tests
addTest(new LinkNodeTest("testInstantiated"));
addTest(new LinkNodeTest("testAutoAddChild"));
addTest(new LinkNodeTest("testManualAddChild"));
addTest( failedTest );
Labels:
actionscript,
code,
efficiency,
frameworks,
object oriented,
workflow
Sunday, June 14, 2009
Typecast slip up in with Numbers in As3
I always seem to run into the same problem with Actionscript 3 when typecasting (converting an object from one type to another). It has to do with the use of the as keyword because I've fallen into the habit of doing all typecasting this way - the benefit being that it will fail gracefully and return the default value for the type you're trying to cast to (usually this is a null).
The trickiness comes when I try to typecast something to a number like this:
var testString:String = "77";
trace( testString as Number );
// Traces null
var testNumber:Number = testString as Number;
trace( testNumber );
// Traces 0
This is a problem because this particular syntax will always return a null, which sometimes leads me to spend too much time tracing this down because sometimes I'm writing code that handles null values gracefully. Anyways, the proper way is:
var testString:String = "77";
trace( Number(testString) );
// Traces 77
Because you're actually utilizing conversion functions for Top Level classes like Number, String, Array, which override casting one might do with the Number(object) kind of syntax when using non-top level classes. These can also lead to unexpected results if you aren't careful.
Labels:
actionscript,
code,
D'OH
Friday, June 12, 2009
The making of Bjork's Wanderlust
I recently came across this behind the scenes look at the Bjork 3d video, Wanderlust. It's by a group named Encyclopedia Pictura, and it's an amazing feat of multidisciplinary creativity (and mushrooms). Some time last year they gave a talk at Horizons - a conference on psychedelic drugs that I arranged some videography for. They incorporate 3d recording and playback, large scale puppetry, clay, choreography, green screening, and 3d compositing to achieve an amazing texture and visual quality.
Wednesday, June 10, 2009
Images on Jet Propulsion Lab site open for use
I spent some time working on a site design for someone where the theme of space was tossed around a bit. I did some research and found that the Jet Propulsion Labs (a branch of NASA) - perhaps because they're publicly funded or something - allow for the open use of images gathered from satellite and robotics missions. There are a few stipulations, but giving someone a credit line for some amazing images seems like a more than fair price to pay. They even have a nice little flex gallery for browsing their collection and offer both high rez Jpgs and Tiffs.
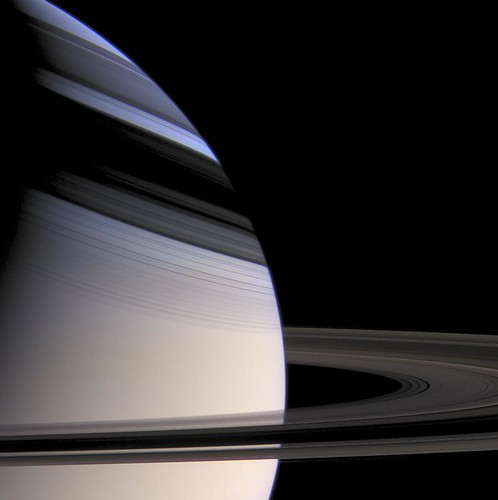
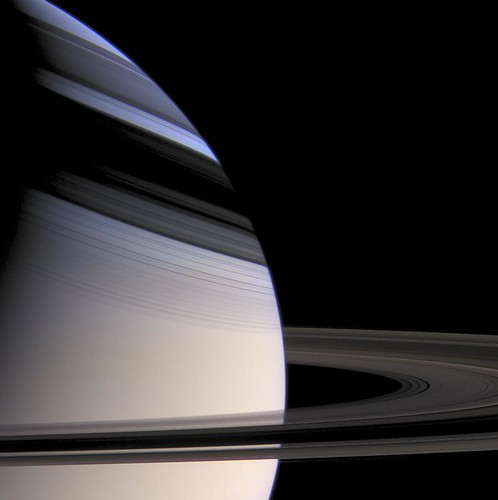
I'm pretty sure that - within these stipulations - an artist would even be able to use these images as source material to photoshop to their hearts content, but rather than give you incorrect legal information here I'll just direct you over to the JPL's Image Use Policy so you can see for yourself :)
Sunday, June 7, 2009
Code package management in flash and eclipse
There was a time where I'd have a copy of a framework or as3 code package in the folder of every project I'd use it in. This wasn't a big deal, until I did a search one day and realized I had about nine copies of Tweener strewn about my harddrive - a situation ridiculous enough to merit change.
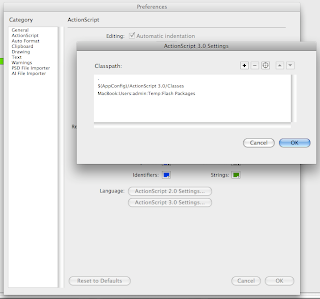
There are also a number of token/variable things you can use to represent certain important locations. If anyone knows of more, let me know:
I created a folder named Flash Packages, and copied every bit of code I reuse (or even might) to it. In the flash IDE the change was simple enough - add this new folder to your list of classpaths. To get there you open Flash's preferences, navigate to the Actionscript panel, and - under the "Language" label - click on the button for Actionscript 2 or 3 respectively. This will alter the global classpath (affecting every flash document you create in the IDE). Settings for the document-level classpath can be found in the Publishing settings. Add your new created and sorted package folder to the list and you're done :)
What's more interesting, however, is the fact that you can add relative paths as well. There's a default entry of "." which causes every FLA to include any classes in it's own directory, while an entry of ".." would cause the directory's parent to be searched.
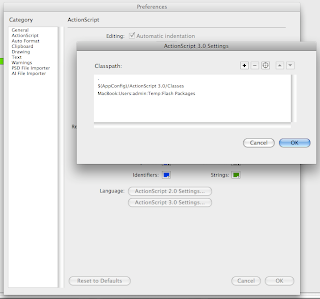
There are also a number of token/variable things you can use to represent certain important locations. If anyone knows of more, let me know:
- $(AppConfig) - Flash CS3 configuration folder, which contains default Actionscript classes
- $(LocalData) - Not exactly sure where this one points
My workflow includes both flash and the Eclipse editor, though, and for the longest time I couldn't figure out how to mirror this kind of change in it's environment. Just found out how to do it, though, and it's pretty easy. Just add a folder to your project (New>Folder), click the Advanced button, and set up the option for "Link To Folder In the File System" :) This is where you'll add packages from the folder of actionscript libraries you created earlier.
Labels:
actionscript,
code,
eclipse,
fdt,
workflow
Web 2.0 Mission Statement
Was clearing out some old papers and came across some ideas I'd doodled on paper while working out the concept for an editing project I did over a year ago where I mixed animated flash content, screen captures of various websites, and live video. Thought it might be cool to share the two together. Make sure you watch it in high quality :)
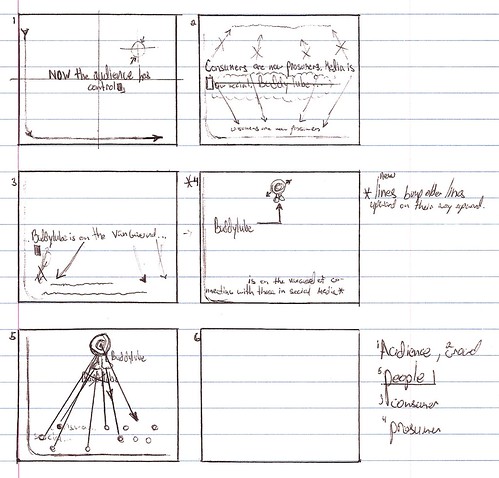
Concept, Editing, Animation - Will Saunders Producer, Copy - Lee McAlilly 3d Animation (Not seen here) - Broadway Romero Additional Editing - Jeltron
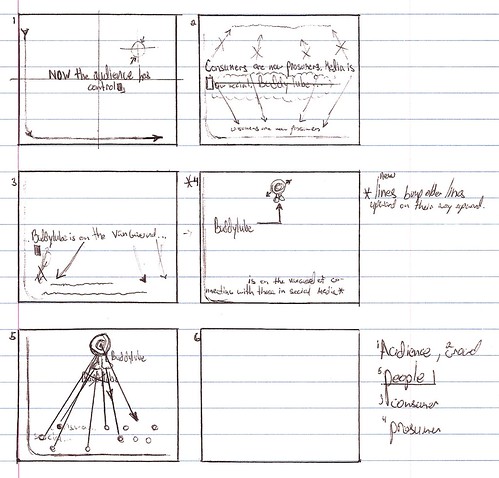
Labels:
mission statement,
post production,
sketchbook,
web2.0
Monday, June 1, 2009
In with the crowd.
Despite a certain article I read on FreelanceSwitch recently about the downfalls of spec work, I've joined a crowd-sourcing site. If you don't know, crowd-sourcing is when a job - normally given to a business or freelancer - is outsourced to a large mass, usually the community of a website. Designers competing for jobs, basically. The writer sites the one I joined as an example, which is run by another blog I read a lot geared towards web designers, and - amazingly enough - explains the head-explodingly meta case of another crowd-sourcing site obtaining their logo in this manner from this one (and ultimately only paying around $200).
This case - as ridiculously ironic as it is - would drive anyone to a strong opinion, but at the end of the day it still stands that everyone involved is a grown-up designer. In other words, this site can't be seen as more than a tool and any tool looks bad when used improperly. The most obvious use case for these sites - in my opinion - is passive income. I, however, choose to see them as an opportunity, if you will. I believe the number of contests requiring logos far outweigh the others, but branding is something I'd like to get (more) into anyways. A number of things will have to be kept in mind if this is to make any kind of sense, however -
- These are little, self-contained, modular experiments in design. I can walk into them open minded and unburdened, and - after the alloted amount of time - leave just the same. I have to remember that ultimately, I'm just here to pimp the system.
- I must manage time. I could be the best logo designer in the world but the cost/risk/payment ratio is so skewed that it wouldn't make sense to spend too much time or rely too heavily on these things. Also consider the fact that my 30 minute idea has gotten a more positive response from the contest holder than two I did for another that took significantly longer.
- What makes these "exercises" particularly potent is the fact that it's an amalgamation of design and branding, so it's not just the photoshop experience I'm after - it's also ability to do this type of high-level conceptual thinking involved with quickness and agility. A terribly beneficial aspect, despite the fact that I would probably outsource this kind of task to someone else myself if a client were to ask for it.
- Though this site won't teach me the value of design, I'll certainly learn a lot by seeing the designs of others and - more importantly - see how ideas can be realized, hybridized, and cannibalized, and where the fine lines in the sand between the three lie. Already I can see familiar concepts applied in new, interesting ways I wouldn't have thought of, examples of people taking my concepts and running with them, and comments from designers to designers about what they consider to be imitations of theirs.
- That ultimately this is supposed to be fun, and that I'm not concerned with the $200 that could - in some cases - not be paid at all if the contest holder doesn't find a design they like. By keeping this in mind I'll be more likely to stop and change direction if it's just not working out or even just keep the designs to myself.
- If I manage to get good at this, I can sell designs on the back hand - through a logo shop or an asset store - a site where people purchase pre-made components for use in their projects.
- It gives me (a) perspective on what people consider a logo. It's important to understand parameters and trends so you can make the decision when to fit your work within their constraints and when (and how) to break/stand out. Speaking of trends, I think this list will cover most of them you'll come across - easily (or unfortunately) enough.
As I said before - logo creation might not be something I'd hesitate to bring in a specialist regarding, but the state of the art has definitely elevated to the point where the website - traditionally a source of relatively static information, now has just as much to do with a brand as a logo - and is indeed the first point of contact people have with both. I suppose this is more of a strategic exercise then, if that's the case.
Thursday, May 21, 2009
Auteur theory (2.0)
Film - though a medium well over one hundred years old - is still riddled with everlasting debate of the Auteur Theory, or the question of whom the creative direction belongs to on a film. A hundreds years is actually pretty young in the context of other cross-disciplinary collaborative arts like architecture. I wonder why - with this debate so common-place in film - is it not as heavily milled over in web-design, a similar but far less ripe medium. The director steers the ship according to the Auteur Theory. What's the equivalent in web design? It's an important question to consider in the face of highly trained artistic teams who craft fully immersive experiences. At this point I'm sure a boutique design agency somewhere has justified the cost of a 35mm film shoot for the sake of a site and a site alone.
What makes this parallel even more interesting is the fact that film (ok ok...video) is undergoing a radical shift in the exact opposite direction - while websites are becoming more complex and members of web-design teams increasingly specialized, the craft of "filmmaking" is now subject to fewer barriers to entry than ever. Cheap(er) "prosumer" cameras with high resolution chips and well-rounded video production software packages make it easier for a single person to manifest a vision. Think of what you get with the Final Cut Suite (editing, compression, titles, scoring) now compared to your cheapest low-end Avid (editing) from years ago. Meanwhile the average flash designer now has to get up on Flex, integration with back-end data services, and have the ability to do 3d math. It's no wonder these teams are becoming more granular, and though perhaps we've boiled the question down a bit this much still stands - is it better to be the lone artist or part of a well oiled machine?
Firstly I wonder about a team's capacity to push the envelope. While more people on a project definitely increase the chances for groupthink, with the right people the process evolves into an iterative collaboration with each person elaborating on the last's ideas until the whole becomes more than the sum of it's parts. I suppose I've always been attracted to the idea of agencies or companies that encourage play, also. Google employees have a certain amount of time they're allowed to devote to projects of their choosing, and a person with the distributed support of a team probably has more time that sort of thing, in theory. Despite all the rationalization in the world, however, this much will always be true - a team of people will have more time than one. If you consider play crucial to the design process (and allot time for it), it only becomes another task weighing on the already limited resources of the freelancer.
But enough talk though - let's make with the practicality:
http://www.insidepiet.com
An amazing site created by one person.
http://in2media.com
An equally killer site that seems to be more of a group output.
The most obvious difference is in the information design. Both sites are essentially an entity's attempt at letting you know what services they offer, and selling you on them. I'd like to think it'd be blaringly obvious from the density of composition and the adherence to common web interface conventions which is which, even if the text here or on the sites didn't obviously state so. In2Media, though they use interesting effects on their visual components and make a bold artistic statement that gives the site cohesiveness with their use of the floating glass illustrations, these things are still secondary to the information. At the end of the day it's still very much a column-driven, moderately text heavy site. With Inside Piet the information takes a serious backseat to frill. This could be a case of function following form, except the function -is- to showcase the form, making it a "look at what I'm capable of" kind of site. Not to downplay it, though, because for him it works - just like the In2Media for them. DesignM.ag has an interview with Matthew Jurmann of Chromatic where freelancing and small business are compared and contrasted.
A designer friend (who I highly respect) and I were having a discussion just the other day about specialization. He'd been learning a little code on the side so that he'd be less dependent on coders, or at the very least more able to understand the parameters when working with them. When I briefly mentioned something about wanting to be the next renaissance man, he told me about another designer at his job in a similar position who ultimately decided that he'd rather focus on design and be great at design instead of spreading himself thin over too many things. While I - regularly feeling more like a jack of all trades (master of none) - can totally see the merit in that, I think nod will always go to the integrator for thinking across multiple disciplines, whether that be one person with polymath-like skills or a team able to effectively brainstorm, delegate, and collaborate. This is something I'll probably be thinking about a lot over the coming weeks and months.
What makes this parallel even more interesting is the fact that film (ok ok...video) is undergoing a radical shift in the exact opposite direction - while websites are becoming more complex and members of web-design teams increasingly specialized, the craft of "filmmaking" is now subject to fewer barriers to entry than ever. Cheap(er) "prosumer" cameras with high resolution chips and well-rounded video production software packages make it easier for a single person to manifest a vision. Think of what you get with the Final Cut Suite (editing, compression, titles, scoring) now compared to your cheapest low-end Avid (editing) from years ago. Meanwhile the average flash designer now has to get up on Flex, integration with back-end data services, and have the ability to do 3d math. It's no wonder these teams are becoming more granular, and though perhaps we've boiled the question down a bit this much still stands - is it better to be the lone artist or part of a well oiled machine?
Firstly I wonder about a team's capacity to push the envelope. While more people on a project definitely increase the chances for groupthink, with the right people the process evolves into an iterative collaboration with each person elaborating on the last's ideas until the whole becomes more than the sum of it's parts. I suppose I've always been attracted to the idea of agencies or companies that encourage play, also. Google employees have a certain amount of time they're allowed to devote to projects of their choosing, and a person with the distributed support of a team probably has more time that sort of thing, in theory. Despite all the rationalization in the world, however, this much will always be true - a team of people will have more time than one. If you consider play crucial to the design process (and allot time for it), it only becomes another task weighing on the already limited resources of the freelancer.
But enough talk though - let's make with the practicality:
http://www.insidepiet.com
An amazing site created by one person.
http://in2media.com
An equally killer site that seems to be more of a group output.
The most obvious difference is in the information design. Both sites are essentially an entity's attempt at letting you know what services they offer, and selling you on them. I'd like to think it'd be blaringly obvious from the density of composition and the adherence to common web interface conventions which is which, even if the text here or on the sites didn't obviously state so. In2Media, though they use interesting effects on their visual components and make a bold artistic statement that gives the site cohesiveness with their use of the floating glass illustrations, these things are still secondary to the information. At the end of the day it's still very much a column-driven, moderately text heavy site. With Inside Piet the information takes a serious backseat to frill. This could be a case of function following form, except the function -is- to showcase the form, making it a "look at what I'm capable of" kind of site. Not to downplay it, though, because for him it works - just like the In2Media for them. DesignM.ag has an interview with Matthew Jurmann of Chromatic where freelancing and small business are compared and contrasted.
A designer friend (who I highly respect) and I were having a discussion just the other day about specialization. He'd been learning a little code on the side so that he'd be less dependent on coders, or at the very least more able to understand the parameters when working with them. When I briefly mentioned something about wanting to be the next renaissance man, he told me about another designer at his job in a similar position who ultimately decided that he'd rather focus on design and be great at design instead of spreading himself thin over too many things. While I - regularly feeling more like a jack of all trades (master of none) - can totally see the merit in that, I think nod will always go to the integrator for thinking across multiple disciplines, whether that be one person with polymath-like skills or a team able to effectively brainstorm, delegate, and collaborate. This is something I'll probably be thinking about a lot over the coming weeks and months.
Labels:
theory
Saturday, May 16, 2009
Zen like design.
fillslashstroke had a good find. The people who made this seriously knocked one out of the park :)
'Green Box' Product Promo (Pizza Box) from Green Box on Vimeo.
Labels:
design-tech useability
Monday, April 20, 2009
Workflow
I'm starting to realize just how big of an undertaking this could all turn out to be. Drastic changes will have to be made if it's going to be anything resembling feasible. Though I see this is a primarily artistic endeavor, efficiency will be key. Hell, especially because it's artistic. It's as easy for an artist to lose all track of time in pursuit of some romantic notion as it is for a programmer to spend several days straight hunched over a screen atop a mountain of empty Mountain Dew cans sans food. If I'm to figure out where I can improve, I'll need a bit more clarity concerning the time I spend on things, and the act of refining takes plenty of it so I'll only be spending more.
Sitepoint was gracious enough to have covered the act of time tracking already. Here's their list of 7 Time Tracking Tools. I'm giving Time Tracker for OSX a try. It's simple and the price is right. Used to have some dashboard widget that had similar functionality but I'd always forget to turn it off. Time tracker gives you a nice little icon in the menu bar.
Anyways, as much time as i'd be recovering through greater insight, there's also time I could be saving through efficiency in planning, attention to detail, and - most important - modularity. To me this sounds like Object Oriented Programming. For those of you that don't know, here's what Wikipedia has to say about it:
Object-oriented programming (OOP) is a programming paradigm that uses "objects" and their interactions to design applications and computer programs. [...] As hardware and software became increasingly complex, quality was often compromised. Researchers studied ways to maintain software quality and developed object-oriented programming in part to address common problems by strongly emphasizing discrete, reusable units of programming logic.
By breaking a program down into modular, reusable components, you increase agility (the ability to change conceptual directions quickly yet w/ stability ) and productivity. It's quite inspiring to see solid Object Oriented code at work. So inspirational that it really just makes common sense to apply it to areas outside of just code. In fact I'm sure there are hundreds of man-made situations where the label fits despite designers not being aware of the concept of things being O.O, not to mention countless examples in science and nature. Where better to draw inspiration from, right?
This paradigm closely reflects the structure of systems 'in the real world', and it is therefore well suited to model complex systems with complex behaviours.Niklaus Wirth - Good Ideas through the Looking Glass
The most obvious application of these tenants is to the code I make for websites so that's where I've began - by doing a great deal of research and planning for an Actionscript 3 framework. I'll spend a lot of time getting the technical details right this once so that - in the future - I'll be able to focus primarily on the overall design of a site. While this will be primarily geared towards sites, it won't take much to expand it with a backend for RIAs (Rich Internet Applications), and I can even see myself going as far as using it in interactive art installations. To get my ideas out in solid but fluid form I used PersonalBrain - a program for "mind mapping." With the right kind of thinking, you can use it to plan out anything that can be thought of in terms of hierarchical or interrelated concepts (which is pretty much everything ever so...). I highly recommend checking it out. Here's a screenshot of the mindmap for the framework thus far:
The entire map is much bigger than what's here but there are the areas I plan on focusing on initially. I've already got quite a few classes in the package so I'll probably be writing about it with more depth soon (and posting source).
I'll be racking my brain over the next few weeks to figure out how to maximize efficiency. Stay tuned.
Labels:
actionscript,
efficiency,
frameworks,
mindmapping,
object oriented,
workflow
Monday, April 13, 2009
An experiment in design
This blog will be an experiment in design - my once unrecognized and unreciprocated infatuation. For long I've admired from afar - obsessing over the production design of movies like Blade Runner and Dune. Spending countless hours collecting, replicating, and cataloguing the details of objects with amazing aesthetic appeal - sometimes things that did not even really exist, like Kaneda's Bike from Akira (but a quick google search will tell you I'm not the only one taken by it's design).
But I am no designer. An aspiring developer, yes. An aspiring editor of video and film as well. I even web design, and though I've been involved in all these outlets for years I can't help but feel like a Jack of all trades (and a master of none).
But that's ok because I'm finally seeing the potential.
Through the deliberate use of visual and narrative elements, movies suspend your disbelief. If viewers must "buy" into this vision then the aesthetic must work to sell. Production design builds on the emotional content of the script to convey a world critical to that emotion.
Coders construct programs - tools that, though built atop complex logical patterns and interlocking "modules", ease the completion of certain tasks. If designed effectively these programs allow users to comprehend parameters of the task without being bogged down by the inner workings of the program (or the task) itself. This level of abstraction is taken a step further. Not only is the task simplified through the "lens" of the program, the program itself is also simplified through the lens of the users preconceptions and emotional bias towards the task. These are the concerns of user interface design.
Design is as much about function as it is about form. As much about the internal as it is the aesthetic.
When I take this into account, I understand my attraction to web design - it fits somewhere complicatedly between the two, but it's the complexities of these connections that make them ripe. I tried to flush out more of these parallels but reading a bunch of blogs and sites about web design will quickly reveal just how new a field it is.
So I started looking backwards - specifically at architecture, another field very much concerned with the balance of the technical and the aesthetic. Of both form and function. This is a parallel of more relevance than you think - in programming, Design Patterns are one of the major tenants of Object Oriented code, but the idea (and the phrase) have origins in architecture. It's these kinds of conceptual bridges I'm after.
Through case studies, exercises, and a fair bit of tinkering and research, I'll - among other things - dissect areas where artistic and technical design intersect gracefully. There are fields that have been around a lot longer than the mediums we work with, but are rich in wisdom, methods, and techniques of relevance to today's designer. The tenants of efficient programming tell you to avoid reinventing the wheel, I'm just trying to figure out what else I can avoid the reinventing by re-contextualizing information from other similarly balanced fields.
I also want to know how many of these paradigms that are normally relegated to one facet can be applied in a wider scope to a project. If my website's code is Object Oriented, what would it look like if the visual design was "Object Oriented"? What if the day to day of your business we're run according to the tenants of OOP? These are just some examples of things I'll be getting into on this blog. I'll also talk about actionscript/flash, graphic/motion design, general programming, branding/corporate identity, frameworks (both programmatic and otherwise), photography, inspiration, post-production, and much much more :)
When a concept intrigues me I'll share all the pertinent information I've found, speculate on how and where else it can be applied, and document my success (or lack thereof) with applying it to the design business I'll be starting, with source code, assets, and explanations all along the way. This blog is an experiment in design and journaling of my attempts at patching up the holes in my skill-set. A guide to others hoping to build bridges, and - hopefully - a resource that'll inspire some valuable dialogue on all related matters. Most importantly it's a place where you'll find free stuff, if you're a designer. We all know how designers love free stuff.
Labels:
film
Subscribe to:
Posts (Atom)